Array Destructuring assignment is a convenient syntax for extracting values from arrays or properties from objects into distinct variables. This shorter syntax enhances code readability and maintainability.
There are two types of destructuring assignment expressions: array destructuring and object destructuring. Let’s explore how each of them works:.
Array Destructuring
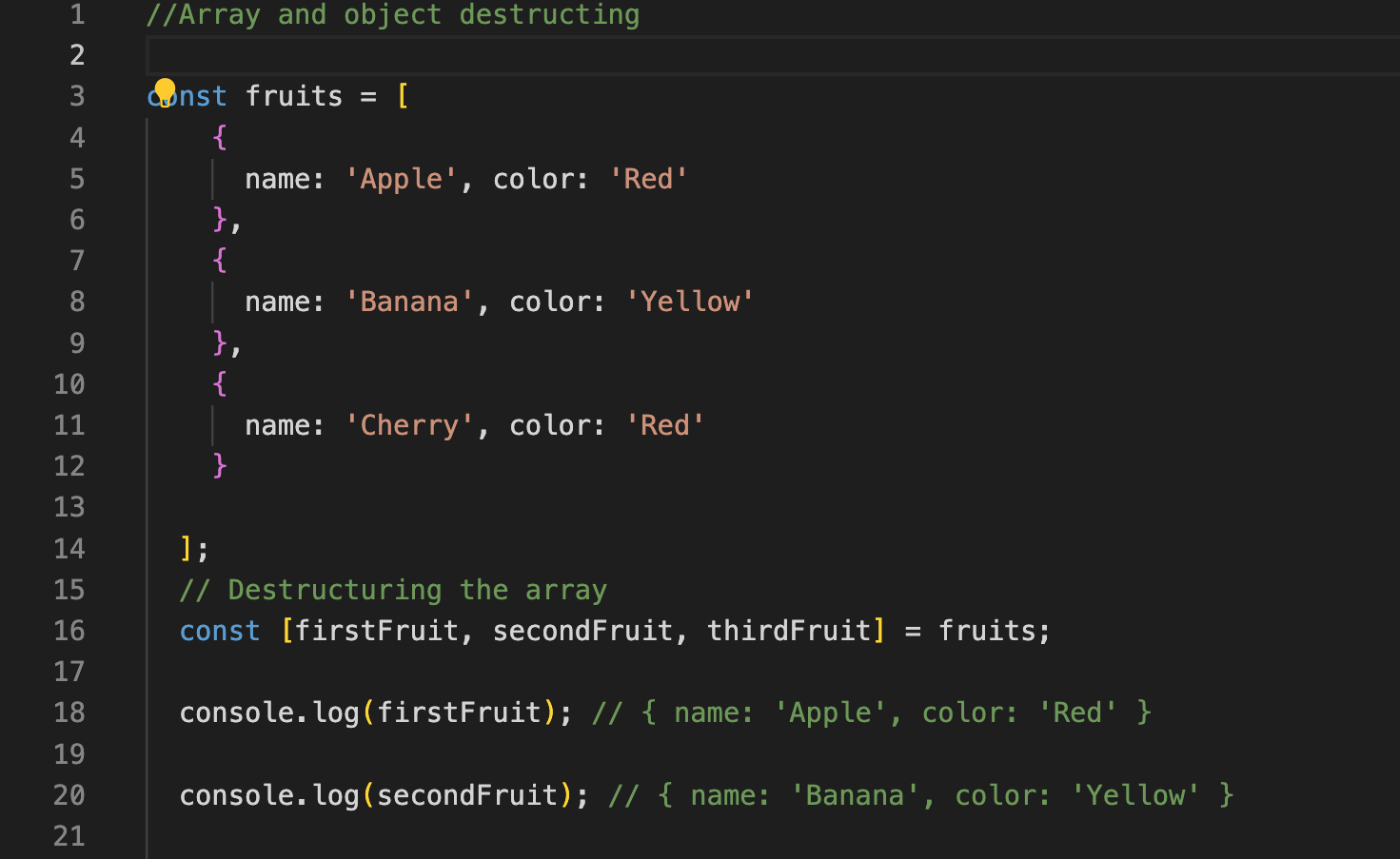
Before ES6, extracting individual values from an array required more verbose code, such as:
Basic Syntax:
ES5 Syntax:
In ES5, you’ll need to manually access properties and array elements:
// Array of fruit objects
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
// Accessing elements manually
var firstFruit = fruits[0];
var secondFruit = fruits[1];
var thirdFruit = fruits[2];
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Array of fruit objects
var fruits = [
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
];
// Accessing elements manually
var firstFruit = fruits[0];
var secondFruit = fruits[1];
var thirdFruit = fruits[2];
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Array of fruit objects
var fruits = [
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
];
// Accessing elements manually
var firstFruit = fruits[0];
var secondFruit = fruits[1];
var thirdFruit = fruits[2];
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Accessing specific properties manually
var firstName = fruits[0].name;
var firstColor = fruits[0].color;
var secondName = fruits[1].name;
var thirdColor = fruits[2].color;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
// Accessing specific properties manually
var firstName = fruits[0].name;
var firstColor = fruits[0].color;
var secondName = fruits[1].name;
var thirdColor = fruits[2].color;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
// Accessing specific properties manually
var firstName = fruits[0].name;
var firstColor = fruits[0].color;
var secondName = fruits[1].name;
var thirdColor = fruits[2].color;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
ES6 Syntax:
In ES6, you can use array and object destructuring to make it more concise:
// Array of fruit objects
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
// Array of fruit objects
const fruits = [
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
];
// Array of fruit objects
const fruits = [
{ name: 'Apple', color: 'Red' },
{ name: 'Banana', color: 'Yellow' },
{ name: 'Cherry', color: 'Red' }
];
// Destructuring the array
const [firstFruit, secondFruit, thirdFruit] = fruits;
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Destructuring the array
const [firstFruit, secondFruit, thirdFruit] = fruits;
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Destructuring the array
const [firstFruit, secondFruit, thirdFruit] = fruits;
console.log(firstFruit); // { name: 'Apple', color: 'Red' }
console.log(secondFruit); // { name: 'Banana', color: 'Yellow' }
console.log(thirdFruit); // { name: 'Cherry', color: 'Red' }
// Destructuring within the destructured objects
const [{ name: firstName, color: firstColor }, { name: secondName }, { color: thirdColor }] = fruits;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
// Destructuring within the destructured objects
const [{ name: firstName, color: firstColor }, { name: secondName }, { color: thirdColor }] = fruits;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
// Destructuring within the destructured objects
const [{ name: firstName, color: firstColor }, { name: secondName }, { color: thirdColor }] = fruits;
console.log(firstName); // 'Apple'
console.log(firstColor); // 'Red'
console.log(secondName); // 'Banana'
console.log(thirdColor); // 'Red'
ES5 syntax
var fruits = ["Apple", "Banana"];
console.log(b); // Banana
var fruits = ["Apple", "Banana"];
var a = fruits[0];
var b = fruits[1];
console.log(a); // Apple
console.log(b); // Banana
var fruits = ["Apple", "Banana"];
var a = fruits[0];
var b = fruits[1];
console.log(a); // Apple
console.log(b); // Banana
In ES6, we can do the same thing in just one line using the array destructuring assignment:
ES6 syntax:
let fruits = ["Apple", "Banana"];
let [a, b] = fruits; // Array destructuring assignment
console.log(b); // Banana
let fruits = ["Apple", "Banana"];
let [a, b] = fruits; // Array destructuring assignment
console.log(a); // Apple
console.log(b); // Banana
let fruits = ["Apple", "Banana"];
let [a, b] = fruits; // Array destructuring assignment
console.log(a); // Apple
console.log(b); // Banana
You can also use rest operator in the array destructuring assignment, as shown here:
ES6 syntax:
let fruits = ["Apple", "Banana", "Mango"];
console.log(r); // Banana,Mango
console.log(Array.isArray(r)); // true
let fruits = ["Apple", "Banana", "Mango"];
let [a, ...r] = fruits;
console.log(a); // Apple
console.log(r); // Banana,Mango
console.log(Array.isArray(r)); // true
let fruits = ["Apple", "Banana", "Mango"];
let [a, ...r] = fruits;
console.log(a); // Apple
console.log(r); // Banana,Mango
console.log(Array.isArray(r)); // true
The object destructuring assignment
In ES5 to extract the property values of an object we need to write something like this:
ES5 syntax
var persons = {name: "Peter", age: 28};
console.log(name); // Peter
var persons = {name: "Peter", age: 28};
var name = persons.name;
var age = persons.age;
console.log(name); // Peter
console.log(age); // 28
var persons = {name: "Peter", age: 28};
var name = persons.name;
var age = persons.age;
console.log(name); // Peter
console.log(age); // 28
But in ES6, you can extract object’s property values and assign them to the variables easily like this:
ES6 syntax
let persons = {name: "Peter", age: 28};
let {name, age} = persons; // Object destructuring assignment
console.log(name); // Peter
let persons = {name: "Peter", age: 28};
let {name, age} = persons; // Object destructuring assignment
console.log(name); // Peter
console.log(age); // 28
let persons = {name: "Peter", age: 28};
let {name, age} = persons; // Object destructuring assignment
console.log(name); // Peter
console.log(age); // 28
Conclusion:
In conclusion, ES6 has fundamentally transformed JavaScript development with its array of powerful features, such as arrow functions, template literals, and block-scoped variables. These advancements have empowered developers to write cleaner, more efficient, and maintainable code, contributing to JavaScript’s ongoing success and adaptability in the ever-evolving landscape of web development.
Thank you for reading, and have a great day!
Also Read:-
git commit -m vs git commit -am
PHP filter_var() Function
Also Visit:-
https://inimisttech.com/